16.1 댓글 보기의 개요
서버에서 처리한 댓글을 사용자가 볼 수 있도록 뷰 페이지 만들고 출력
게시판의 특정 글을 클릭하면 상세 페이지(show)가 뜨고 그 아래에 댓글(_comments)이 보임
댓글 영역
기존 댓글을 보여주는 영역(_list)
새 댓글을 입력하는 영역(_new)00
두 영역은 별도의 뷰 페이지를 만들어 상세 페이지 아래에 삽입하는 형태로 구현
16.2 댓글 뷰 페이지 삽입하기
http://localhost:8080/articles/5
이 화면 아래에 댓글을 출력하도록 함
controller/ArticleController.java
@GetMapping("/articles/{id}")
public String show(@PathVariable Long id, Model model){
log.info("id = " + id);
// 1. id를 조회해 DB에서 해당 데이터 가져오기
Article articleEntity = articleRepository.findById(id).orElse(null);
// 2. 가져온 데이터를 모델에 등록하기
model.addAttribute("article", articleEntity);
// 3. 조회한 데이터를 사용자에게 보여 주기 위한 뷰 페이지 만들고 반환하기
return "articles/show";
}
show() 메서드에서 return 문을 보았을 때 반환하는 뷰 페이지가 articles 디렉터리의 show.mustache 파일임을 확인
templates/articles/show.mustache
<a href="/articles">Go to Article List</a>
{{>comments/_comments}} <!-- 상세 페이지에 댓글 뷰 파일 삽입 -->
{{>layouts/footer}}
푸터 바로 위에 댓글 뷰 파일 삽입({{>comments/_comments}})

빌드 후, 웹 페이지를 새로고침하면 아직_comments.mustache 파일을 만들지 않아 에러페이지가 뜸
templates에서 comments 디렉터리 생성
comments에 _comments.mustache 파일 생성

파일 생성 후, 망치 아이콘을 클릭하고 웹 페이지를 새로 고침하면 에러 페이지가 나오지 않음
templates/comments/_comments.mustache
<div>
<!-- 댓글 목록 보기 -->
{{>comments/_list}}
<!-- 새 댓글 작성하기 -->
{{>comments/_new}}
</div>
<div></div> 태그로 레이아웃을 잡고 2개 영역으로 나누어 뷰 파일 삽입
_list.mustache 파일: 댓글 목록을 보여줌
_new.mustache 파일: 새 댓글을 작성
두 파일 모두 comments 디렉터리에 존재
_list.mustache 파일과 _new.mustache 파일을 만들지 않은 상태로 망치 아이콘을 다시 클릭하고 새로 고침하면
파일이 없기때문에 에러 페이지가 뜸
comments 디렉터리에 _list.mustache 파일과 _new.mustache 파일 생성
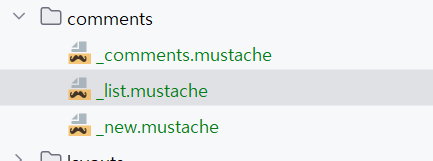
templates/comments/_list.mustache
<div id="comments-list">
{{#commentDtos}}
<div class="card m" id="comments-{{id}}">
<div class="card-header">
{{nickname}}
</div>
<div class="card-body">
{{body}}
</div>
</div>
{{/commentDtos}}
</div>
<div></div> 태그로 댓글 목록 전체를 보여주는 영역을 만들고 id="comments-list"로 설정
조회한 댓글 목록에서 댓글을 하나씩 꺼내 순회할 수 있도록 {{#commentDtos}} ... {{/commentDtos}} 추가
(commentDtos가 여러 데이터라면 머스테치 문법 안쪽에 있는 내용을 반복하라는 뜻)
<div></div> 태그로 댓글 하나를 보여주는 영역을 만듦
class="card" 속성과 id="comments-{{id}}" 속성 추가
각각 해당 영역을 카드 구조로 만들라는 뜻,
commentsDtos에 있는 id 값을 삽입해 해당 영역의 id를 comments-1, comments-2, comments-3 ... 로 설정하라는 뜻
<div></div> 태그로 댓글 내에서 헤더 영역과 본문 영역 만듦
카드의 헤더에서는 commentDtos에 담겨 있는 {{nickname}}을 보여 줌
카드의 본문에서는 commentDtos에 담겨 있는 {{body}}를 보여 줌

에러 페이지가 뜨진 않지만 아무런 댓글이 보이지 않음
→ 뷰 페이지에서 사용하는 commentDtos를 모델에 등록하지 않았기 때문
16.3 댓글 목록 가져오기
뷰 페이지에서 사용할 댓글 목록을 모델에 등록하고 화면에 출력하도록 함
controller/ArticleController.java
public class ArticleController {
@Autowired
private ArticleRepository articleRepository;
@Autowired
private CommentService commentService;
(중략)
@GetMapping("/articles/{id}")
public String show(@PathVariable Long id, Model model){
log.info("id = " + id);
// 1. id를 조회해 DB에서 해당 데이터 가져오기
Article articleEntity = articleRepository.findById(id).orElse(null);
List<CommentDto> commentsDtos = commentService.comments(id);
// 2. 가져온 데이터를 모델에 등록하기
model.addAttribute("article", articleEntity);
model.addAttribute("commentDtos", commentsDtos);
// 3. 조회한 데이터를 사용자에게 보여 주기 위한 뷰 페이지 만들고 반환하기
return "articles/show";
}
(중략)
}
CommentService의 comments(id) 메서드를 호출하여 조회한 댓글 목록을 List<CommentsDto> 타입의 commentDtos 변수에 저장
CommentService 객체 주입하여 컨트롤러에서 CommentService를 사용할 수 있게 함
받아 온 댓글 목록(CommentDtos)을 모델에 등록
서버 재시작 후 http://localhost:8080/articles/5 페이지 새로고침
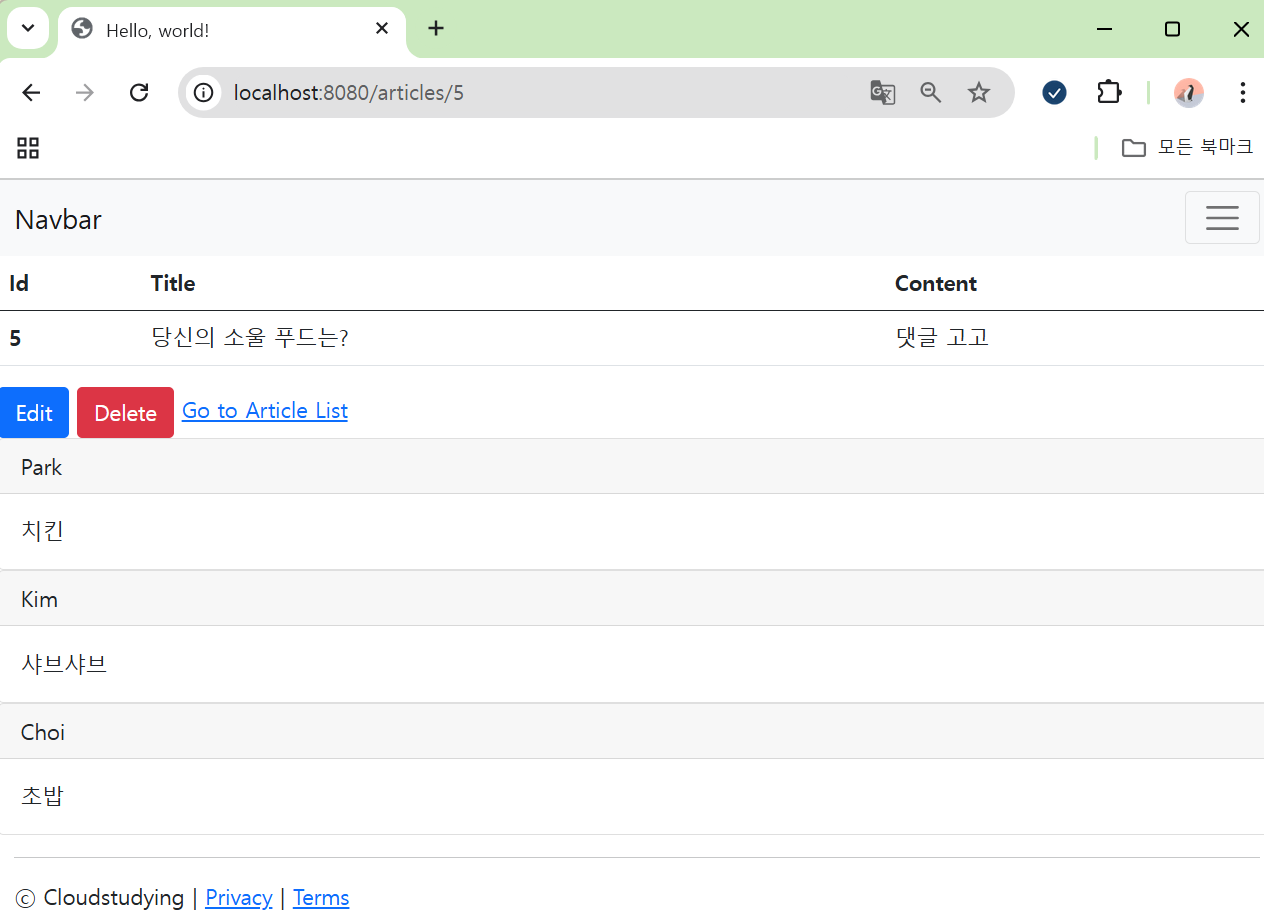
댓글 간격 보기 좋게 벌어지도록 수정
templates/comments/_list.mustache
<div id="comments-list">
{{#commentDtos}}
<div class="card m-2" id="comments-{{id}}">
<div class="card-header">
{{nickname}}
</div>
<div class="card-body">
{{body}}
</div>
</div>
{{/commentDtos}}
</div>
카드 요소의 마진(margin), 바깥 여백을 2만큼 줌
"card m-2"로 수정
< 수정 후 >
'백엔드 > 코딩 자율학습 스프링 부트 3 자바 백엔드 개발 입문 1~2장' 카테고리의 다른 글
15장. 댓글 컨트롤러와 서비스 만들기 (0) | 2025.01.17 |
---|---|
14장. 댓글 엔티티와 리파지터리 만들기 (0) | 2025.01.11 |
13장. 테스트 코드 작성하기 (0) | 2025.01.11 |
12장. 서비스 계층과 트랜잭션 (0) | 2025.01.11 |
5장. 게시글 읽기: Read (0) | 2024.12.21 |